Possible Charged Particle Field
4 posters
Page 3 of 15
Page 3 of 15 • 1, 2, 3, 4 ... 9 ... 15
Re: Possible Charged Particle Field
Using Vectors Efficiently
The length of a vector is calculated on the basis of Pythagoras' theorem. Therefore, it is calculated using the square of each side. To find the length of a given vector, we first calculate the square of its length and then take the square root of that value. The square root operation is expensive, and should be avoided if at all possible, so most vector classes will give you 2 methods: one to retrieve the actual length of the vector; and another to retrieve the square of the length.
There are times when you absolutely need the length, so use it and forget about all of this. However, if you are not really using the length, say you only want to compare it to another vector, then you can just compare the squared lengths of each vector because the square of A is always less than the square of B if A is less than B.
The THREE.Vector3 class provides the lengthSquared method to retrieve the squared length. I haven't looked, but I imagine that the length function would look something like this:
- Code:
THREE.Vector3.prototype.length = function()
{
return Math.sqrt( this.lengthSquared() );
};
So you can avoid that Math.sqrt call by using lengthSquared directly.
When you do need the actual length, or any calculated value really, then store it in a variable if you use it more than once. Otherwise you are calculating it over and over again which is just wasting time and every microsecond counts in a 3D system.
First: get it working.
Second: optimize it to work efficiently.
Eventually you start to optimize while you are getting it working, just because you become familiar with what you need to do, but don't stress about things like this while you are getting something off the ground. I only mention this because I saw some of the new code was inefficient, so it seemed like a good time for a little lesson.
Re: Possible Charged Particle Field
After some testing I have found that my theory is wrong. It is not entering the collision code multiple times for the same collision. The problem is that the second collision, during the same frame, calculates a resultant velocity twice the size of the first.
Suppose we had only 2 particles. We loop over them to determine current interactions and find that they are touching, so we enter the collision code for particle 1, P1, being collided with by particle 2, P2. This calculates the correct velocity. After that, we enter the collision code for P2 being collided with by P1, ie, the other side of the same collision. This calculates a velocity twice the size of the first when it should just calculate an equal and opposite velocity.
I'm still tracking down where the problem is but wanted to let you know in case you were getting frustrated.
Suppose we had only 2 particles. We loop over them to determine current interactions and find that they are touching, so we enter the collision code for particle 1, P1, being collided with by particle 2, P2. This calculates the correct velocity. After that, we enter the collision code for P2 being collided with by P1, ie, the other side of the same collision. This calculates a velocity twice the size of the first when it should just calculate an equal and opposite velocity.
I'm still tracking down where the problem is but wanted to let you know in case you were getting frustrated.
Re: Possible Charged Particle Field
Ok, just fixed it. I have pushed the changes to GIT.
The problem was that it was calculating the new velocity rather than the change in velocity. Therefore it would increase the energy of the collisions as it went because it didn't remove the existing velocity from the change which was then added to the existing velocity, hence doubling it each collision.
The problem was that it was calculating the new velocity rather than the change in velocity. Therefore it would increase the energy of the collisions as it went because it didn't remove the existing velocity from the change which was then added to the existing velocity, hence doubling it each collision.
Re: Possible Charged Particle Field
I also removed all of that repositioning code, since it is not needed, and reverted the optimizedPA calculation to use 2.0 instead of 4.0, which set it back to the original value. We now get a nice transfer of momentum.
Re: Possible Charged Particle Field
Don't feel bad about missing this one. It can be tricky figuring out exactly what the math is doing. I imposed some constraints on you, i.e. I wanted a change in velocity, but most systems don't work that way because they only care about the velocity and nothing else. We have a situation where we have other forces to apply, so we can't just calculate the new velocity and be done with it. We have to add it in with other velocities from other forces.
When you first start out developing apps, you tend to search, copy and refine other peoples code. Hell, I still do that at times, as do all developers. As you become more familiar with the concepts you are dealing with, in this case the math, you start to work at that level rather than the code. You start to see how to manipulate things to how you want them to work, rather than working the way someone else wanted their app to work.
My goal, ever since you showed interest in learning to program, has been to get you to that level. To feel familiar enough with the code to start to think more about the physics. I want you to be able to take some rough idea, hammer it into a concept and be able to see how you might implement that in code to show others your ideas, or just to create a playground for yourself.
It's a long path, with lots of math, but you can get there. You're already well on the way.
When you first start out developing apps, you tend to search, copy and refine other peoples code. Hell, I still do that at times, as do all developers. As you become more familiar with the concepts you are dealing with, in this case the math, you start to work at that level rather than the code. You start to see how to manipulate things to how you want them to work, rather than working the way someone else wanted their app to work.
My goal, ever since you showed interest in learning to program, has been to get you to that level. To feel familiar enough with the code to start to think more about the physics. I want you to be able to take some rough idea, hammer it into a concept and be able to see how you might implement that in code to show others your ideas, or just to create a playground for yourself.
It's a long path, with lots of math, but you can get there. You're already well on the way.
Re: Possible Charged Particle Field
.
Hey Nevyn, I haven't reviewed your latest changes yet. I'm delighted you found a problem or two. Going to the optimizedPA value of 2.0 is a relief.
On the other hand, I believe it was a mistake for you to remove re-positioning the particle centers. Here's a picture explaining why.
Do you think there's anything wrong with this image?

I'm certain that repositioning is necessary for higher velocities.
.
Hey Nevyn, I haven't reviewed your latest changes yet. I'm delighted you found a problem or two. Going to the optimizedPA value of 2.0 is a relief.
On the other hand, I believe it was a mistake for you to remove re-positioning the particle centers. Here's a picture explaining why.
Do you think there's anything wrong with this image?

I'm certain that repositioning is necessary for higher velocities.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
You are using the wrong unit distance in both of those calculations. You are ignoring the normalization of the vector by doing so. After normalization, the length of both vectors are now 1 and that length is what becomes the unit distance. Both a1 and a2 are calculated after normalization, so they always see a unit distance of 1. Therefore, the values for a1 and a2 do not change as a result of overlapping.
Let's think about it a different way and I'll use your comments in the code as my source.
This means we want to find the direction from one particle to the other. Imagine you are that particle, this vector tells you what direction you need to look in order to look directly at the other particle. This is the direction that force is being applied.
It is normalized because we don't care about the distance between these particles. We only care about the direction. The reason for this is more about efficiency than the rules of math. If we know that we are using a normalized vector, then we don't have to calculate its length or include it in the math if we can avoid it. In this case, that vector is going to be scaled to the force of the collision so we need it to be of length 1 for that to work correctly.
This is the important piece of the puzzle, because this is the calculation of a1 and a2. I think that you are missing a key part of this sentence. It is actually about 2 different things so it will be easier if I split it and add in a join:
Find the length of each particles motion
(but we only want the motion)
along the linear component CPA
This means that we want to find the length of the existing velocity, for each particle, that is in the same direction as the line between their centers, which we found earlier. We don't care about the velocity component in other directions (that are orthogonal to this one) so we extract just the amount of velocity that is along that vector.
It is the length of the existing velocity that we want to find and we are only using the line between their centers to provide a coordinate system. That line does not provide length, only direction.
The dot product is a way to find the length of one vector, along the direction of another vector. It tells you how much of one vector is expressed in the direction of the other.
An example might help to see what I mean.
Suppose we have 2 particles with a radius of 1. P1 is at (0, 0, 0) and P2 is at (4, 5, 0). Let's give P2 a velocity of (-1, -1, 0) which means that after 4 seconds, the bottom of P2 will collide with the top of P1. At that point in time, P2 will be directly above P1.
The line between their center will be (0, 2, 0) - (0, 0, 0) = (0, 2, 0).
Normalized this becomes (0, 1, 0).
Now we want to find the component of the existing velocity that is along that line. We will ignore P1 because it doesn't have any existing velocity. P2 has a velocity of (-1, -1, 0) but we only want the component along (0, 1, 0) which will be (0, -1, 0).
We are ignoring the X dimension because the collision is happening in the Y dimension. We don't care how much velocity is in the X or Z dimensions so we get rid of them. This is the same thing that I did in the charge point calculations. I split the vectors up into components so that I could use them in different ways. In this case, it is being used to remove the velocity that does not effect the collision. Because the force is along the Y dimension, we don't want any X or Z velocity being used.
Let's think about it a different way and I'll use your comments in the code as my source.
Airman wrote:
// Find the normalized vector between the centers of the
// overlapping receiver and emitter particles.
This means we want to find the direction from one particle to the other. Imagine you are that particle, this vector tells you what direction you need to look in order to look directly at the other particle. This is the direction that force is being applied.
It is normalized because we don't care about the distance between these particles. We only care about the direction. The reason for this is more about efficiency than the rules of math. If we know that we are using a normalized vector, then we don't have to calculate its length or include it in the math if we can avoid it. In this case, that vector is going to be scaled to the force of the collision so we need it to be of length 1 for that to work correctly.
Airman wrote:
// Find the length of each particle's motion along the linear
// component CPA.
This is the important piece of the puzzle, because this is the calculation of a1 and a2. I think that you are missing a key part of this sentence. It is actually about 2 different things so it will be easier if I split it and add in a join:
Find the length of each particles motion
(but we only want the motion)
along the linear component CPA
This means that we want to find the length of the existing velocity, for each particle, that is in the same direction as the line between their centers, which we found earlier. We don't care about the velocity component in other directions (that are orthogonal to this one) so we extract just the amount of velocity that is along that vector.
It is the length of the existing velocity that we want to find and we are only using the line between their centers to provide a coordinate system. That line does not provide length, only direction.
The dot product is a way to find the length of one vector, along the direction of another vector. It tells you how much of one vector is expressed in the direction of the other.
An example might help to see what I mean.
Suppose we have 2 particles with a radius of 1. P1 is at (0, 0, 0) and P2 is at (4, 5, 0). Let's give P2 a velocity of (-1, -1, 0) which means that after 4 seconds, the bottom of P2 will collide with the top of P1. At that point in time, P2 will be directly above P1.
The line between their center will be (0, 2, 0) - (0, 0, 0) = (0, 2, 0).
Normalized this becomes (0, 1, 0).
Now we want to find the component of the existing velocity that is along that line. We will ignore P1 because it doesn't have any existing velocity. P2 has a velocity of (-1, -1, 0) but we only want the component along (0, 1, 0) which will be (0, -1, 0).
We are ignoring the X dimension because the collision is happening in the Y dimension. We don't care how much velocity is in the X or Z dimensions so we get rid of them. This is the same thing that I did in the charge point calculations. I split the vectors up into components so that I could use them in different ways. In this case, it is being used to remove the velocity that does not effect the collision. Because the force is along the Y dimension, we don't want any X or Z velocity being used.
Re: Possible Charged Particle Field
.
Thanks Nevyn. I'll have you know I'm really enjoying this project, and learning a lot.
Most importantly I appreciate your patience and knowledge and cooperation. I apologize for being too defensive or aggressive at times.
My only regret is for the poor readers. Are they bored or what?
.
Thanks Nevyn. I'll have you know I'm really enjoying this project, and learning a lot.
Most importantly I appreciate your patience and knowledge and cooperation. I apologize for being too defensive or aggressive at times.
My only regret is for the poor readers. Are they bored or what?
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
No need for apologies. Arguing your case is the only way to learn how strong it is. And the only way for me to see what you are missing or for me to find out that I am missing something. You are in a difficult position at the moment because you are fairly new to programming and trying to juggle the physics and the coding is a lot to handle. You're doing fine.
Re: Possible Charged Particle Field
It's not boring, just a bit over my head! A lot over my head. I'm trying to absorb it all too so appreciate you two sharing it all here - perhaps I can use it in my own stuff at some point, to "teach" or program Maya to behave how I want it to.
Meanwhile, do you plan on making a visual simulation with this coding? Is it something we can look at when things are tuned and working? If not that's fine, I'm just such a visual person it might be helpful.
And of course, defining and understanding the workings of charge ever better is the ultimate goal and key here for me. You are both fundamental in this stuff. Miles is often a bit too short with me to be terribly helpful, but I imagine he just wants people to do their own damn work and let him do his.
Meanwhile, do you plan on making a visual simulation with this coding? Is it something we can look at when things are tuned and working? If not that's fine, I'm just such a visual person it might be helpful.
And of course, defining and understanding the workings of charge ever better is the ultimate goal and key here for me. You are both fundamental in this stuff. Miles is often a bit too short with me to be terribly helpful, but I imagine he just wants people to do their own damn work and let him do his.

Jared Magneson- Posts : 525
Join date : 2016-10-11
Re: Possible Charged Particle Field
Yeah, we will get something out there at some point. Right now, we are just throwing it through as many different scenarios as we can come up with to make sure that the math is working.
I'm not really sure how we are going to make this app user friendly. It is an engine, not an app, really. It is the kind of thing where you setup the initial conditions and it runs through the resulting interactions. I'm not sure how to let the user setup the initial conditions. Maybe I could create a little scripting language for the user to describe the particles, their positions, orientations, velocities and spins. That gets pretty complicated. I don't know. I'm open to suggestions.
I'm not really sure how we are going to make this app user friendly. It is an engine, not an app, really. It is the kind of thing where you setup the initial conditions and it runs through the resulting interactions. I'm not sure how to let the user setup the initial conditions. Maybe I could create a little scripting language for the user to describe the particles, their positions, orientations, velocities and spins. That gets pretty complicated. I don't know. I'm open to suggestions.
Re: Possible Charged Particle Field
I don't know if you need to worry about user input yet, I mean it sounds like a noble cause and a great goal. I'm just curious what it looks like! Can you output or record an animation of it? I'm way behind here so if my request sounds stupid, I apologize.
And obviously no rush. Just showing some interest.
And obviously no rush. Just showing some interest.

Jared Magneson- Posts : 525
Join date : 2016-10-11
Re: Possible Charged Particle Field
I have updated the version I put up on my site so that it presents a menu containing all of the scenarios that are available. Select one and it will reload the page and execute that scenario.
https://www.nevyns-lab.com/mathis/app/cpim/test.html
https://www.nevyns-lab.com/mathis/app/cpim/test.html
Re: Possible Charged Particle Field
.
Wow Nevyn. Given Jared’s request I thought you were working on CPIM. Making test.html available with gui selection greatly exceeded my expectations. Given test's availability, I feel compelled to spend more time to clean up, add and/or improve a few scenarios.
Jared, I’d be more than happy to implement any configuration you suggest - for example, set up views and such, draft with gifs, add the best to scenarios for the full blown implementation. By the way, how do you make videos? A few days ago this site wouldn’t accept my gif. I went to Vimeo thinking they had some video maker available but I didn’t see one.
We need gravity next. We cannot observe orbital behavior without it.
.
Wow Nevyn. Given Jared’s request I thought you were working on CPIM. Making test.html available with gui selection greatly exceeded my expectations. Given test's availability, I feel compelled to spend more time to clean up, add and/or improve a few scenarios.
Jared, I’d be more than happy to implement any configuration you suggest - for example, set up views and such, draft with gifs, add the best to scenarios for the full blown implementation. By the way, how do you make videos? A few days ago this site wouldn’t accept my gif. I went to Vimeo thinking they had some video maker available but I didn’t see one.
We need gravity next. We cannot observe orbital behavior without it.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Yippee! This is good stuff.
The RandomBody experiment is my favorite so far.
The RandomBody experiment is my favorite so far.
Jared Magneson- Posts : 525
Join date : 2016-10-11
Re: Possible Charged Particle Field
Airman, have a look at the changes I made to test.html. I have restructured the init*Body* functions so that they all belong to an object (called Scenarios). The menu is dynamically generated based on the functions defined in that object, so you can just add more and they will be included in the menu automatically.
I did try to get the function names to look better in the menu, but it didn't work out like I wanted, so it is what it is. Good enough for now.
I think I will add a way to group functions into folders so that the menu is a bit more streamlined. If we add more functions it will get too long to be workable. That doesn't mean that you shouldn't add new ones, please do, I'll have the new menu working soon enough.
I did try to get the function names to look better in the menu, but it didn't work out like I wanted, so it is what it is. Good enough for now.
I think I will add a way to group functions into folders so that the menu is a bit more streamlined. If we add more functions it will get too long to be workable. That doesn't mean that you shouldn't add new ones, please do, I'll have the new menu working soon enough.
Re: Possible Charged Particle Field
I've pushed some changes to GIT with the new menu grouping functionality. I also created a few new scenarios for proton stacks. Made the random function more versatile and added a couple of new scenarios for it. They basically set the chance of the particles having a velocity and/or orientation. Random01 has 0% chance of velocity and 25% chance of an orientation. Random02 has 25% velocity, 50% orientation. Random03 has 50% velocity, 75% orientation.
Re: Possible Charged Particle Field
.
Ok, I cleaned up lattice03, then created lattice04 - a 15x20 grid - and was amazed and horrified by the array of collision errors revealed. It didn't matter, fast or slow, some percentage of collisions were degrees of awful.
I was more surprised to find I cleared those errors by removing (commenting out) point1 and point2.
I take it back. We need those points. Those horrible collision bugs are real.
.
Ok, I cleaned up lattice03, then created lattice04 - a 15x20 grid - and was amazed and horrified by the array of collision errors revealed. It didn't matter, fast or slow, some percentage of collisions were degrees of awful.
I was more surprised to find I cleared those errors by removing (commenting out) point1 and point2.
- Code:
///vectorCPA.copy(point1);
///vectorCPA.sub(point2);
I take it back. We need those points. Those horrible collision bugs are real.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Sorry I haven't gotten back to you about this one, Airman, I've been out-of-commission the last few days with a bad cold. I've had a bit of a look but nothing is jumping out at me (and it would have to in my current state).
I tried to adjust point1 and point2 used to calculate the line between the particle centers by subtracting their existing velocity from their position to find their last position (although the velocities used are far too large for that, because they are in real world units like m/s, not in per frame units that we would need, but since it is normalized, it shouldn't matter) but it didn't help. My theory was the velocities were too large so a particle could move past another one before the collision was processed.
That didn't really make much sense because not every collision gets messed up. Only the odd one seems to get a bit stuck. It isn't even consistent with which ones get stuck. For example, you might run it and the 4th particle in the top row gets into trouble, but on another run, that line is fine and the 3rd from the top might fail. Looks like it is one of those horrible inconsistent errors that are very hard to track down. My guess is that the data is getting corrupted at some point. Sometimes 2 particles can get stuck together for some time and if you zoom in on them, they are twitching. Eventually, they separate with a too large velocity (because it has been building during all of those stuck together collisions).
We need to figure out how the particles are getting stuck together. After the change in velocity has been calculated, check the length of that vector and compare it to the length of the existing velocity vector to see if it is larger. If it is, then something is wrong (in this scenario, not all). Try something like this:
...
// we only need to check v2 because v1 should be 0
if( v.length() > v2.length() )
{
debugger;
}
If you have the debugging window open at the time (press F12 in Firerox and I think Chrome, maybe even IE/Edge) then it will stop at that point and you can see what all of the variables are and hopefully see something that doesn't fit. Look closely at the positions and existing velocities to see if they contain any invalid data. Look at the direction of vectorCPA, in this scenario it should always be in the positive X direction. Maybe add this code just after the normalization of vectorCPA:
if( vectorCPA.x != 1 )
{
debugger;
}
That will catch anything wrong with the line between their centers.
I tried to adjust point1 and point2 used to calculate the line between the particle centers by subtracting their existing velocity from their position to find their last position (although the velocities used are far too large for that, because they are in real world units like m/s, not in per frame units that we would need, but since it is normalized, it shouldn't matter) but it didn't help. My theory was the velocities were too large so a particle could move past another one before the collision was processed.
That didn't really make much sense because not every collision gets messed up. Only the odd one seems to get a bit stuck. It isn't even consistent with which ones get stuck. For example, you might run it and the 4th particle in the top row gets into trouble, but on another run, that line is fine and the 3rd from the top might fail. Looks like it is one of those horrible inconsistent errors that are very hard to track down. My guess is that the data is getting corrupted at some point. Sometimes 2 particles can get stuck together for some time and if you zoom in on them, they are twitching. Eventually, they separate with a too large velocity (because it has been building during all of those stuck together collisions).
We need to figure out how the particles are getting stuck together. After the change in velocity has been calculated, check the length of that vector and compare it to the length of the existing velocity vector to see if it is larger. If it is, then something is wrong (in this scenario, not all). Try something like this:
...
// we only need to check v2 because v1 should be 0
if( v.length() > v2.length() )
{
debugger;
}
If you have the debugging window open at the time (press F12 in Firerox and I think Chrome, maybe even IE/Edge) then it will stop at that point and you can see what all of the variables are and hopefully see something that doesn't fit. Look closely at the positions and existing velocities to see if they contain any invalid data. Look at the direction of vectorCPA, in this scenario it should always be in the positive X direction. Maybe add this code just after the normalization of vectorCPA:
if( vectorCPA.x != 1 )
{
debugger;
}
That will catch anything wrong with the line between their centers.
Re: Possible Charged Particle Field
.
The good news, I’ve been working on the charge field. Trying to make a little progress every day.
The first order of business here is to show some of these awful collision errors. To make that easier, I added an XY-grid to my previous collision lattice in order to show where the colliding neutrons should end up. The particles on the leftmost edge are given different starting velocities to the right. 10 at the bottom, 15, 20, 25, 30, 35, 40, and 45 at the top. If the particle does not end up on an XY intersection, it was affected by a collision error.

This gif shows four sets of collisions: a,b,c, and d - sorry they’re not labeled.
Overlapping particles. Each of the four sets has at least a pair of overlapping particles. The third set – c - has two. a:1, b:1, c:2, d:1. Overlapped particles can remain together for some time, they may separate at the highest velocities observed while also causing negative velocities - collisions traveling to the left..
Pushing particles. When two particles meet they stay together for a while. They may separate quickly or turn into overlapped particles. Pushed particles usually end up to the right of their XY finish coordinates.
Feel free to comment.
The bad news, I’m experiencing GIT errors. I did not forget my password. I'm unable to push.
Nevyn, if you'd be so kind, help! I see you just posted, sorry you've got a bug.
.
The good news, I’ve been working on the charge field. Trying to make a little progress every day.
The first order of business here is to show some of these awful collision errors. To make that easier, I added an XY-grid to my previous collision lattice in order to show where the colliding neutrons should end up. The particles on the leftmost edge are given different starting velocities to the right. 10 at the bottom, 15, 20, 25, 30, 35, 40, and 45 at the top. If the particle does not end up on an XY intersection, it was affected by a collision error.

This gif shows four sets of collisions: a,b,c, and d - sorry they’re not labeled.
Overlapping particles. Each of the four sets has at least a pair of overlapping particles. The third set – c - has two. a:1, b:1, c:2, d:1. Overlapped particles can remain together for some time, they may separate at the highest velocities observed while also causing negative velocities - collisions traveling to the left..
Pushing particles. When two particles meet they stay together for a while. They may separate quickly or turn into overlapped particles. Pushed particles usually end up to the right of their XY finish coordinates.
Feel free to comment.
The bad news, I’m experiencing GIT errors. I did not forget my password. I'm unable to push.
: Invalid username or password. If your organization manages your account or you've enabled two-step verification, create an app password to log in:
Nevyn, if you'd be so kind, help! I see you just posted, sorry you've got a bug.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Not sure if it is related, but I saw that you have 2 BitBucket accounts when I gave you access to this project. Maybe you are using the other one without realising it? I did something like that between my personal account and my work account.
Go to the BitBucket web page and login there. A few weeks ago I had to do that at work because SourceTree wouldn't login for me. After logging in to the webpage, it started to work again.
Go to the BitBucket web page and login there. A few weeks ago I had to do that at work because SourceTree wouldn't login for me. After logging in to the webpage, it started to work again.
Re: Possible Charged Particle Field
.
I logged into Bitbucket first with sourcetree off - what I usually do - twice in the last 20 minutes, no joy.
I think you're right, Bitbucket is telling me to create a log-in to Sourcetree, so I'll see about doing that.
I'll try again tomorrow, then send you a pm to let you know.
.
I logged into Bitbucket first with sourcetree off - what I usually do - twice in the last 20 minutes, no joy.
I think you're right, Bitbucket is telling me to create a log-in to Sourcetree, so I'll see about doing that.
I'll try again tomorrow, then send you a pm to let you know.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
It is a bit hard to tell exactly, but in your GIF above, it looks like when 2 particles get stuck together, they move along with about half of the velocity of the original moving particle, until they separate. That could be an important clue. What would cause the calculated velocity to be half of what it should be? Although I have seen 2 particles stuck together that were not moving, apart from twitching until they separate.
Re: Possible Charged Particle Field
.
Yes, I noticed that stuck particles are moving at less than the 'initial' velocity, I'm not sure if it's half or not. I guess the resultant motion is initial motion minus some of the reaction, but the reaction is insufficient to separate the two particles.
Thanks for the debugging clues and the console points. I'll give it a shot.
.
Yes, I noticed that stuck particles are moving at less than the 'initial' velocity, I'm not sure if it's half or not. I guess the resultant motion is initial motion minus some of the reaction, but the reaction is insufficient to separate the two particles.
Thanks for the debugging clues and the console points. I'll give it a shot.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Given the equation being used:
var optimizedPA = (2.0 * (a1 - a2))/(m1 + m2);
We know that m1 and m2 are constants, so they will not change. Therefore, only a1 and a2 can be causing the problems. This means that the calculation of vectorCPA is incorrect, in some circumstances, or that the existing velocities are already corrupt. They are the only things used to calculate a1 and a2. Print some info about them to the console and see if anything looks suspect.
Can you reproduce the problem with only a single line of neutrons? Having so many makes it difficult to step through the code or to interpret console output. It doesn't seem like the number of neutrons should matter, but it would be interesting if it did.
var optimizedPA = (2.0 * (a1 - a2))/(m1 + m2);
We know that m1 and m2 are constants, so they will not change. Therefore, only a1 and a2 can be causing the problems. This means that the calculation of vectorCPA is incorrect, in some circumstances, or that the existing velocities are already corrupt. They are the only things used to calculate a1 and a2. Print some info about them to the console and see if anything looks suspect.
Can you reproduce the problem with only a single line of neutrons? Having so many makes it difficult to step through the code or to interpret console output. It doesn't seem like the number of neutrons should matter, but it would be interesting if it did.
Re: Possible Charged Particle Field
.
I've re-tried a 9 particles in a row about 24X earlier today without seeing any overlapped particles. I don't recall ever seeing a single row with overlapped particles. I DO however, see plenty of pushed particles - particles to the right of their finish marks - occur in single rows. There's no evidence that particles have been pushed using my console settings today. I just saw velocity transfers.
I tried two rows next. 18 particles: two rows of nine particles. Both initial velocities equal 30. 20 or so times also without observing overlap. Again, I may see slightly pushed particles.

24 particles: pOne - pEight. Three rows, velocities 10,20,and 30. Finally saw two overlapped particles, shown in the gif above. Particles three and four almost entirely overlap, but separate before both lost their velocities. Particle 5 picked up the same velocity but it pushed particle 6.

Here's an image showing that pushed particle 6.
I submitted a customer service work ticket with bitbucket about twelve hours ago.
Tried using the debugger after normalization, but it would always stop at the very first collision encountered. How to make an exception? So I commented it out.
I trimmed the console output to just the top row's velocity changes. I haven't figured it out yet. The console output follows. The mix of velocity magnitudes of .999s or round integers suggest there may be an easy ( float v.sub( v1 );///? fix but I don't see a direct link between 999s, integer like #s and pushes.
.
Can you reproduce the problem with only a single line of neutrons? Having so many makes it difficult to step through the code or to interpret console output. It doesn't seem like the number of neutrons should matter, but it would be interesting if it did.
I've re-tried a 9 particles in a row about 24X earlier today without seeing any overlapped particles. I don't recall ever seeing a single row with overlapped particles. I DO however, see plenty of pushed particles - particles to the right of their finish marks - occur in single rows. There's no evidence that particles have been pushed using my console settings today. I just saw velocity transfers.
I tried two rows next. 18 particles: two rows of nine particles. Both initial velocities equal 30. 20 or so times also without observing overlap. Again, I may see slightly pushed particles.

24 particles: pOne - pEight. Three rows, velocities 10,20,and 30. Finally saw two overlapped particles, shown in the gif above. Particles three and four almost entirely overlap, but separate before both lost their velocities. Particle 5 picked up the same velocity but it pushed particle 6.

Here's an image showing that pushed particle 6.
I submitted a customer service work ticket with bitbucket about twelve hours ago.
Tried using the debugger after normalization, but it would always stop at the very first collision encountered. How to make an exception? So I commented it out.
I trimmed the console output to just the top row's velocity changes. I haven't figured it out yet. The console output follows. The mix of velocity magnitudes of .999s or round integers suggest there may be an easy ( float v.sub( v1 );///? fix but I don't see a direct link between 999s, integer like #s and pushes.
- Code:
///////////////////////// Collision between particles 1-2
//// pOne velocity lost. From v1=30, v2=0.
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
//// pTwo velocity increase. v1=0, v2=30.
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///////////////////////// Collision between particles 2-3
//// pTwo velocity lost. From v1=30, v2=0.
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -29.999999999999996
cdm.js:769 a2: 0
cdm.js:806 v: -29.999999999999993, 0, 0
//// pThree velocity increase. v1=0, v2=30.
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 29.999999999999996
cdm.js:806 v: 29.999999999999993, 0, 0
///////////////////////// Collision between particles 3-4
//// pThree velocity lost. From v1=30, v2=0.
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
//// pFour velocity increase. v1=0, v2=30.
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///Pattern break.
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -29.999999999999996
cdm.js:806 v: 29.999999999999993, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 29.999999999999996
cdm.js:769 a2: 0
cdm.js:806 v: -29.999999999999993, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -29.999999999999996
cdm.js:806 v: 29.999999999999993, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 29.999999999999996
cdm.js:769 a2: 0
cdm.js:806 v: -29.999999999999993, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: -30
cdm.js:806 v: 30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: 30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///////////////////////// Collision between particles 4-5
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///////////////////////// Collision between particles 5-6
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///////////////////////// Collision between particles 6-7
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -30
cdm.js:769 a2: 0
cdm.js:806 v: -30, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 30
cdm.js:806 v: 30, 0, 0
///////////////////////// Collision between particles 7-8
cdm.js:746 v1: 30, 0, 0
cdm.js:747 v2: 0, 0, 0
cdm.js:768 a1: -29.999999999999996
cdm.js:769 a2: 0
cdm.js:806 v: -29.999999999999993, 0, 0
cdm.js:746 v1: 0, 0, 0
cdm.js:747 v2: 30, 0, 0
cdm.js:768 a1: 0
cdm.js:769 a2: 29.999999999999996
cdm.js:806 v: 29.999999999999993, 0, 0
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Don't worry about those 29.99999999996 type values. They are just rounding errors in the math. Nothing we can do about it that does not involve more expensive operations (by using higher precision numbers). They are annoying, and they will leave residual velocities where there shouldn't be, but they will be so small as to be unnoticeable unless you just leave it sitting there for a really long time and eventually notice that a particle you thought wasn't moving, has actually moved. They certainly aren't causing our problem.
All of that console output looks fine. Did you actually see an error happen while that output was being generated? I really need to see it when there is an error.
All of that console output looks fine. Did you actually see an error happen while that output was being generated? I really need to see it when there is an error.
Re: Possible Charged Particle Field
All of that console output looks fine. Did you actually see an error happen while that output was being generated? I really need to see it when there is an error.
Correct, although that output isn't fine, it's filled with many additional velocity changes, the twitches you observed in overlapped particles. The gif and console output are from the same collision error event which I observed. I still have it on my browser. I was grateful to find such a compact event. All other collision errors console outputs I observed were much longer, 1 or 2 thousand lines. It's remarkable that all such overlapping events I observed involved the same velocities - just the initial velocities from that row.
I'll try to find a collision error that involves a velocity different from the initial row velocity tomorrow.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
I don't see an errors in that output. Can you point out what you see?
Re: Possible Charged Particle Field
.
Sorry I wasn't clear. An eight particle row without any collision errors results in 7 collisions with a total of 14 velocity changes. In the console output above, I inserted some commentary. The error involves the 36 velocity changes after "Collision between particles 3-4"... "pattern break" and "Collision between particles 4-5". All those velocity changes involve 30, 0 and -30 velocities. I suppose unequal numbers of those velocities may add up to fractional speeds, but I don't see how particles can move faster than just those velocities, so that's the error case I'll look for tomorrow.
.
Sorry I wasn't clear. An eight particle row without any collision errors results in 7 collisions with a total of 14 velocity changes. In the console output above, I inserted some commentary. The error involves the 36 velocity changes after "Collision between particles 3-4"... "pattern break" and "Collision between particles 4-5". All those velocity changes involve 30, 0 and -30 velocities. I suppose unequal numbers of those velocities may add up to fractional speeds, but I don't see how particles can move faster than just those velocities, so that's the error case I'll look for tomorrow.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
I don't think that is correct. The output will follow the algorithm. Add another console.log at the start of the calculateCollision method that writes the index1 and index2 values for you. Then you know which particles are interacting.
console.log( 'P1: ' + index1 + ', P2: ' + index2 );
console.log( 'P1: ' + index1 + ', P2: ' + index2 );
Re: Possible Charged Particle Field
Basically, the twitching happens across multiple frames, never within the same frame as your interpretation of the output would suggest.
Re: Possible Charged Particle Field
Unless those are the only colliding particles, then you would be correct. I don't know right now. It is suggesting that the change in velocity is not being added to the position of the particles under some circumstances. I'll have a look into that code tonight.
Re: Possible Charged Particle Field
No luck.
I didn't find any problems with the addition of velocities or updating the position with that velocity. I added in some console.logs to make sure that things were executing as I thought they should be, and they were. I then decided to re-write the algorithm without using the internal memory buffers, so that it would just retrieve the position, orientation, velocity and spin from the Particle object itself (which will be faster), but that didn't help either.
The main reason I did that was because the memory buffers use a Float32Array object, which are 32bit floats, not 64bit floats, and it might be a number precision problem. It wasn't. I will probably factor that code in anyway, just for the performance gain and I don't think we can use shaders in the way that I wanted to so they are not needed. I keep finding conflicting information about using shaders and don't know what to believe at the moment.
I'm nearly at the point where I want to use a different collision algorithm.
I didn't find any problems with the addition of velocities or updating the position with that velocity. I added in some console.logs to make sure that things were executing as I thought they should be, and they were. I then decided to re-write the algorithm without using the internal memory buffers, so that it would just retrieve the position, orientation, velocity and spin from the Particle object itself (which will be faster), but that didn't help either.
The main reason I did that was because the memory buffers use a Float32Array object, which are 32bit floats, not 64bit floats, and it might be a number precision problem. It wasn't. I will probably factor that code in anyway, just for the performance gain and I don't think we can use shaders in the way that I wanted to so they are not needed. I keep finding conflicting information about using shaders and don't know what to believe at the moment.
I'm nearly at the point where I want to use a different collision algorithm.
Re: Possible Charged Particle Field
With respect to your SourceTree issues, have you updated the app? Does it need an update? There is a flag in the top right corner of the app (on Windows, Mac may be different) and if it is orange, then it wants you to look at it. Sometimes it is just some question but it may be an update notification. I think there were some major updates recently and they required me to login in via the website before SourceTree would login again.
Re: Possible Charged Particle Field
.
WARNING. A lot of ugly follows. Nevyn, you don't sound like you're enjoying yourself, maybe you shouldn't look.

Collision error. Below, A 2X5 collision grid is shown, with particles left to right P0 – P4 on the bottom row, and P5 – P9 on the top - complying with their actual index numbers as referred to in the console output. The horizontal distance between particles is 10, vertical separation is 7.5. P0 is given an initial velocity, v = 30. The top row’s leftmost particle, P5 is given an initial v = 40. The error collision – overlapped particles - occurred in the top row. The bottom row contains 3 pushed particles – a problem which doesn’t seem to be indicated by the current console output. The image below is self-explanatory. The above gif and following closeup details show just the center portion of the top row – the area is shown by the yellow rectangle.
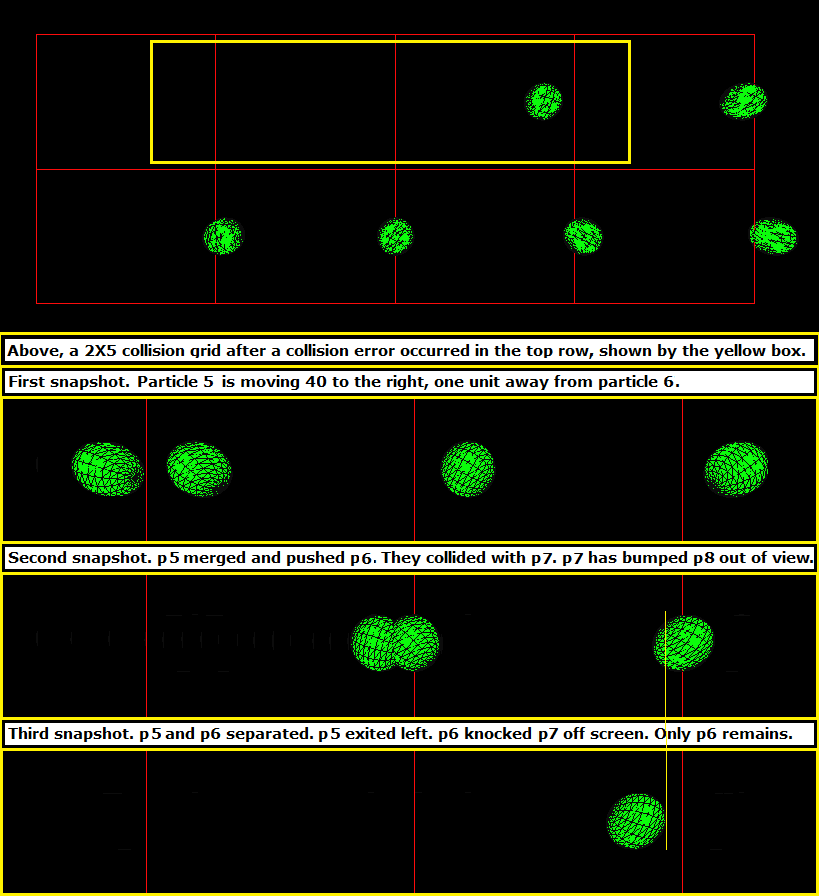
The particle numbers have been changed from my previous descriptions on order to agree with the console output. The thin yellow line at the bottom right shows that p2 pushed p3 before the velocity transfers occurred.
Note that p5 slides under p6 about halfway before p6 joins in and movs to the right. P7/P8 looks to be the one clean collision that occurred in the top row. Three of the four particles in the second row are pushed.
I’ll post the Console output in the next post or two.
.
WARNING. A lot of ugly follows. Nevyn, you don't sound like you're enjoying yourself, maybe you shouldn't look.

Collision error. Below, A 2X5 collision grid is shown, with particles left to right P0 – P4 on the bottom row, and P5 – P9 on the top - complying with their actual index numbers as referred to in the console output. The horizontal distance between particles is 10, vertical separation is 7.5. P0 is given an initial velocity, v = 30. The top row’s leftmost particle, P5 is given an initial v = 40. The error collision – overlapped particles - occurred in the top row. The bottom row contains 3 pushed particles – a problem which doesn’t seem to be indicated by the current console output. The image below is self-explanatory. The above gif and following closeup details show just the center portion of the top row – the area is shown by the yellow rectangle.
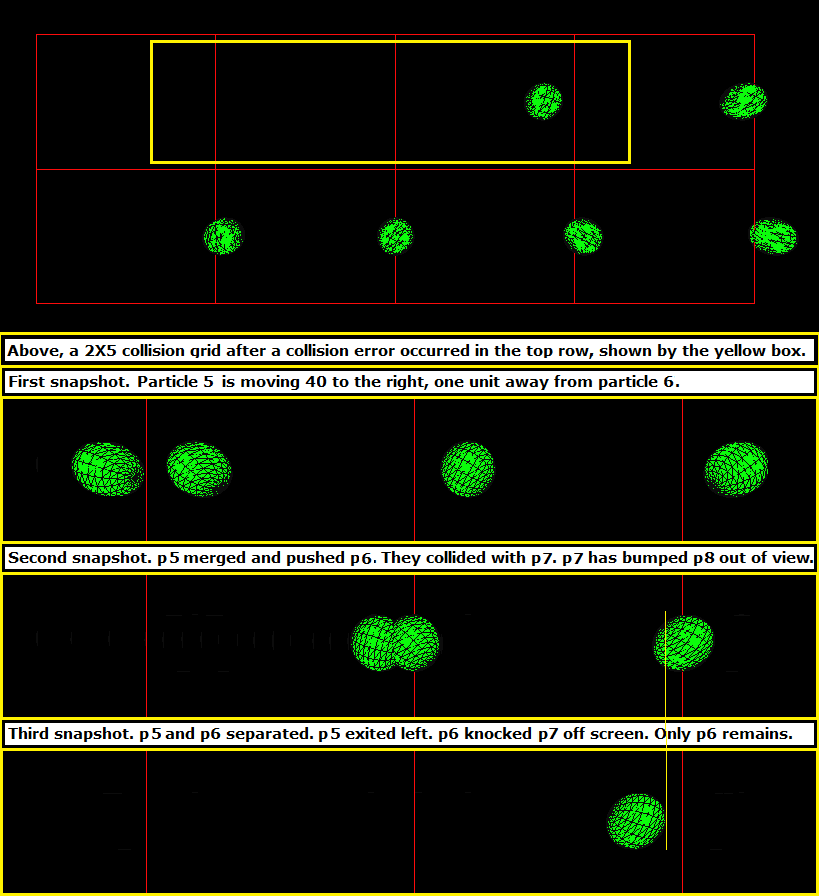
The particle numbers have been changed from my previous descriptions on order to agree with the console output. The thin yellow line at the bottom right shows that p2 pushed p3 before the velocity transfers occurred.
Note that p5 slides under p6 about halfway before p6 joins in and movs to the right. P7/P8 looks to be the one clean collision that occurred in the top row. Three of the four particles in the second row are pushed.
I’ll post the Console output in the next post or two.
.
Last edited by LongtimeAirman on Thu Aug 09, 2018 10:19 pm; edited 1 time in total
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
.
Console output. Each row of 5 particles should result in just 4 collisions, (5-1); times 2 rows, for a total of eight collisions. Given the output variables required at every collision: v1, v2, P1, P2, a1, a2, and v, every non-error collision requires 12 console output lines. We would expect 8*12 or 96 output lines for 8 error free collisions. In this case, row 2 collisions make up 48 lines of the console output. The total number of lines, after removing the line 1 three,js, from the top the console output is exactly 1,000. That would be equal to 1000/12 = 83.33 collisions. That’s my rough summary, I no doubt have details wrong. I concentrated on presentation, I also need to study it. For example; Why is P1: 6, P2: 7 the first collision, shouldn’t it be P1: 5 and P2: 6?? I’ll place a copy of the console output into my GitFolder, but I guess it won’t be pushed for some time. So, without further ado.
I hope you'll be requesting position locations next?
Thanks for that Sourcetree idea, I'll look into it.
.
Console output. Each row of 5 particles should result in just 4 collisions, (5-1); times 2 rows, for a total of eight collisions. Given the output variables required at every collision: v1, v2, P1, P2, a1, a2, and v, every non-error collision requires 12 console output lines. We would expect 8*12 or 96 output lines for 8 error free collisions. In this case, row 2 collisions make up 48 lines of the console output. The total number of lines, after removing the line 1 three,js, from the top the console output is exactly 1,000. That would be equal to 1000/12 = 83.33 collisions. That’s my rough summary, I no doubt have details wrong. I concentrated on presentation, I also need to study it. For example; Why is P1: 6, P2: 7 the first collision, shouldn’t it be P1: 5 and P2: 6?? I’ll place a copy of the console output into my GitFolder, but I guess it won’t be pushed for some time. So, without further ado.
- Code:
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 6, P2: 7
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 7, P2: 6
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 40
cdm.js:807 v: 40, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 7, P2: 8
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 8, P2: 7
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 40
cdm.js:807 v: 40, 0, 0
cdm.js:746 P1: 2, P2: 3
cdm.js:747 v1: 30, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -30
cdm.js:770 a2: 0
cdm.js:807 v: -30, 0, 0
cdm.js:746 P1: 3, P2: 2
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 30, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 30
cdm.js:807 v: 30, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 8, P2: 9
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 9, P2: 8
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 40
cdm.js:807 v: 40, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 3, P2: 4
cdm.js:747 v1: 30, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -30
cdm.js:770 a2: 0
cdm.js:807 v: -30, 0, 0
cdm.js:746 P1: 4, P2: 3
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 30, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 30
cdm.js:807 v: 30, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 3, P2: 4
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 30, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: -30
cdm.js:807 v: 30, 0, 0
cdm.js:746 P1: 4, P2: 3
cdm.js:747 v1: 30, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: 30
cdm.js:770 a2: 0
cdm.js:807 v: -30, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 3, P2: 4
cdm.js:747 v1: 30, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -29.999999999999996
cdm.js:770 a2: 0
cdm.js:807 v: -29.999999999999993, 0, 0
cdm.js:746 P1: 4, P2: 3
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 30, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 29.999999999999996
cdm.js:807 v: 29.999999999999993, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -39.99999999999999
cdm.js:770 a2: 39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -39.99999999999999
cdm.js:770 a2: 39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -39.99999999999999
cdm.js:770 a2: 39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -39.99999999999999
cdm.js:770 a2: 39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: -79.99999999999997, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 39.99999999999999
cdm.js:770 a2: -39.99999999999999
cdm.js:807 v: 79.99999999999997, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: -40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 5, P2: 6
cdm.js:747 v1: -40, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: 80, 0, 0
cdm.js:746 P1: 6, P2: 5
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: -40, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 40
cdm.js:807 v: -80, 0, 0
cdm.js:746 P1: 5, P2: 7
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -39.99999999999999
cdm.js:770 a2: 0
cdm.js:807 v: -39.999999999999986, 0, 0
cdm.js:746 P1: 7, P2: 5
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 39.99999999999999
cdm.js:807 v: 39.999999999999986, 0, 0
cdm.js:746 P1: 7, P2: 8
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 8, P2: 7
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 40
cdm.js:807 v: 40, 0, 0
cdm.js:746 P1: 7, P2: 8
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: -40
cdm.js:807 v: 40, 0, 0
cdm.js:746 P1: 8, P2: 7
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: 40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 7, P2: 8
cdm.js:747 v1: 40, 0, 0
cdm.js:748 v2: 0, 0, 0
cdm.js:769 a1: -40
cdm.js:770 a2: 0
cdm.js:807 v: -40, 0, 0
cdm.js:746 P1: 8, P2: 7
cdm.js:747 v1: 0, 0, 0
cdm.js:748 v2: 40, 0, 0
cdm.js:769 a1: 0
cdm.js:770 a2: 40
cdm.js:807 v: 40, 0, 0
I hope you'll be requesting position locations next?
Thanks for that Sourcetree idea, I'll look into it.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
I am thinking about changing the way these functions are called. They are currently implemented to look for a collision that has just occurred but I want to change it to look for collisions that are about to occur.
Unfortunately, that is a bit difficult. The problem is that I have specified velocities in world units, such as m/s (the seconds is what matters), and I adjust the actual velocity used for a given frame by using the amount of time since the last frame. So if 1/60th of a second has past since the last frame, then I use 1/60th of the velocity. This has the advantage of using the correct amount of velocity for the given time (even if it was 1/45th of a second or 1/73.563). To look for a collision that might happen, I need to know the actual velocity being used, not the real world value, because I need to project into the future to see what will happen.
I just need to find a way around that. I will probably need to pass in the frame time to these methods to do so.
There probably isn't much point to you debugging this issue. Of course, it's a great exercise in finding problems and it really makes you figure out what the code is really doing and why, so you can continue if you want.
I really appreciate the effort you have put in. Also for pushing when you weren't happy with my responses. I haven't been thinking clearly, as my conflicting posts show, so it has been a saving-grace that you haven't just accepted whatever I say or just sat back and waited for me to fix it. Even if I am correct, you need to understand why I am correct and the same thing applies to me. We aren't just building an application, the real important stuff is transferring information and skills, and you have not let yourself down by being a passive student.
Unfortunately, that is a bit difficult. The problem is that I have specified velocities in world units, such as m/s (the seconds is what matters), and I adjust the actual velocity used for a given frame by using the amount of time since the last frame. So if 1/60th of a second has past since the last frame, then I use 1/60th of the velocity. This has the advantage of using the correct amount of velocity for the given time (even if it was 1/45th of a second or 1/73.563). To look for a collision that might happen, I need to know the actual velocity being used, not the real world value, because I need to project into the future to see what will happen.
I just need to find a way around that. I will probably need to pass in the frame time to these methods to do so.
There probably isn't much point to you debugging this issue. Of course, it's a great exercise in finding problems and it really makes you figure out what the code is really doing and why, so you can continue if you want.
I really appreciate the effort you have put in. Also for pushing when you weren't happy with my responses. I haven't been thinking clearly, as my conflicting posts show, so it has been a saving-grace that you haven't just accepted whatever I say or just sat back and waited for me to fix it. Even if I am correct, you need to understand why I am correct and the same thing applies to me. We aren't just building an application, the real important stuff is transferring information and skills, and you have not let yourself down by being a passive student.
Re: Possible Charged Particle Field
.
I saw that flag in Sourcetree. Click on it and it says- Tell us what you think. So I ignored it since. This time I saw that yes, a sourcetree update was available, so I did. After restarting I tried to Push and experienced the same error as before. No joy.
I repeat, this is a great learning opportunity. Frame breaking isn't that difficult, but I'm nowhere near understanding how you've got this application wired else I'd have given you even more grief. I need to study it, even if you don't end up using it. As far as ideas go, I don't like the particle charge emission range = 10. You might consider a charge range limit, based on an angular limit - like the moon and the sun are apparently the same size. Monitor distance^2 just within smaller or closer groups. Or what about gravity? Just messin' with ya.
I admit, part of my effort is to motivate you. You've held up easily, doing a huge amount of work I'm barely able to appreciate. Relax and get your thoughts in order.
.
I saw that flag in Sourcetree. Click on it and it says- Tell us what you think. So I ignored it since. This time I saw that yes, a sourcetree update was available, so I did. After restarting I tried to Push and experienced the same error as before. No joy.
I repeat, this is a great learning opportunity. Frame breaking isn't that difficult, but I'm nowhere near understanding how you've got this application wired else I'd have given you even more grief. I need to study it, even if you don't end up using it. As far as ideas go, I don't like the particle charge emission range = 10. You might consider a charge range limit, based on an angular limit - like the moon and the sun are apparently the same size. Monitor distance^2 just within smaller or closer groups. Or what about gravity? Just messin' with ya.
I admit, part of my effort is to motivate you. You've held up easily, doing a huge amount of work I'm barely able to appreciate. Relax and get your thoughts in order.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
I did exactly the same thing with that orange flag, until a colleague pointed out that it had an update in it.
After updating SourceTree, go to the BitBucket website and login there. Then restart SourceTree and see if you can push. Also, re-enter your password to SourceTree, just in case it has lost it (Tools -> Options -> Authentication tab).
I agree about that emission range. It is a temporary approximation and will be replaced by a 1/r^4 type calculation. Some of the charge interaction code will need to change for the same reason. It is just a rough approximation at the moment and can be made better now that we know the rest of the algorithm works. The current charge code is a linear progression as you move further away from the emitter, which is clearly not right. I was focused on the equator to pole relationship of charge emission and how to process that, so the actual charge calculations were just rough calculations to get something close to what I wanted.
I did some research into the proper math and ran into a problem, which also applies to gravity at this level. Miles' work on that stuff is based on planets and moons. We are working with protons and other tiny particles. Miles takes some time to explain that the 1/r^2 relationship of gravity is actually an outcome of time/distance separation. It takes more time for the charge from the Sun to reach Saturn than it does the Earth, therefore at the exact same moment, Saturn sees older charge (which came from a smaller Sun) than the Earth does. We do not have such time/distance separations and I need to know how to remove such assumptions from the equations.
After updating SourceTree, go to the BitBucket website and login there. Then restart SourceTree and see if you can push. Also, re-enter your password to SourceTree, just in case it has lost it (Tools -> Options -> Authentication tab).
I agree about that emission range. It is a temporary approximation and will be replaced by a 1/r^4 type calculation. Some of the charge interaction code will need to change for the same reason. It is just a rough approximation at the moment and can be made better now that we know the rest of the algorithm works. The current charge code is a linear progression as you move further away from the emitter, which is clearly not right. I was focused on the equator to pole relationship of charge emission and how to process that, so the actual charge calculations were just rough calculations to get something close to what I wanted.
I did some research into the proper math and ran into a problem, which also applies to gravity at this level. Miles' work on that stuff is based on planets and moons. We are working with protons and other tiny particles. Miles takes some time to explain that the 1/r^2 relationship of gravity is actually an outcome of time/distance separation. It takes more time for the charge from the Sun to reach Saturn than it does the Earth, therefore at the exact same moment, Saturn sees older charge (which came from a smaller Sun) than the Earth does. We do not have such time/distance separations and I need to know how to remove such assumptions from the equations.
Re: Possible Charged Particle Field
.
Hey Nevyn, you may have noticed I Pushed to Bitbucket. Customer Service recommended I install a windows bug fix Git Credential Manager for Windows 1.17.0 and remove all bitbucket credentials from my Windows Credential Manager. It worked!
I'm looking at separation distances for the collision errors and was aghast at the overlap distances - for non-error collisions too. The easiest fix may be improved collision detection. I wonder if I could import the console output into excel or R and do this sort of thing automatically.
Tracking the time necessary for separation distances fixes the scale of particle interaction. I agree, a larger scale requires more time. I would assume we have a constant scale for any given particle configuration.Whether we simulate proton or planet, we are adjusting time differentials to account for the motions that we are creating. Sorry if I'm missing the gist.
.
Hey Nevyn, you may have noticed I Pushed to Bitbucket. Customer Service recommended I install a windows bug fix Git Credential Manager for Windows 1.17.0 and remove all bitbucket credentials from my Windows Credential Manager. It worked!
I'm looking at separation distances for the collision errors and was aghast at the overlap distances - for non-error collisions too. The easiest fix may be improved collision detection. I wonder if I could import the console output into excel or R and do this sort of thing automatically.
Tracking the time necessary for separation distances fixes the scale of particle interaction. I agree, a larger scale requires more time. I would assume we have a constant scale for any given particle configuration.Whether we simulate proton or planet, we are adjusting time differentials to account for the motions that we are creating. Sorry if I'm missing the gist.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
Yeah, that's basically it. A planet is a long distance from its star, so it takes time for the charge to reach that planet, even at the extreme speed that charge moves at. But it takes virtually no time for a charge photon to move from one proton to another if they are close enough to be interacting. So we don't need to include the distance separation in that way.
I have been playing with numbers again, and I think I see a way to calculate the charge strengths we want. Instead of a 1/r^4 calculation, it will be a 1/r^3 based equation. That is because it only uses the reduction in volume associated with that distance and not the time, which would bring in the distance again and give us r^4.
Volume of a Sphere: (4*Pi*r^3)/3
A radius of 1 represents the boundary of the emitter. That is the highest charge strength or charge density, which ever way you want to look at it.
A radius of 10 represents the furthest point away from the emitter in this dataset. Note that the radius is from the center of the emitter, not the boundary.
What we are looking at here is how and why the charge diminishes with distance. The particle only emits a certain amount of charge, at any given time or over some set time period, and that is constant (for our purposes here, it can change if the ambient field density changes but would still be a constant for us as we are working relative to the ambient field). So as that charge moves away from the particle, it inhabits a larger volume. The same amount of charge in a greater volume means less density.
So we want to look at how that volume changes as we use a greater radius. You can perform a little bit of calculus on it and find that the 3rd derivative, which is the constant derivative, has a value of 25.1327412287. That doesn't really tell us anything though. So let's take some ratios and see what it tells us.
Firstly, we will divide each volume by the first volume to see how many of the initial volume can fit into the larger volumes. In essence, this is making all volumes relative to the first volume.
A few of those numbers look familiar, and a quick analysis shows that each value is r^3. That wasn't too surprising, since we are using an r^3 equation, but I thought it worth showing as a stepping stone to the next part of this analysis.
That number tells us how much charge we could fit into each volume if we wanted to keep the charge density constant. But we don't want to keep the density constant, we want to keep the amount of charge constant and allow the density to change. So we are looking for the inverse of that value. We want to know how a given amount of charge will fit into a larger volume so we want to divide the first volume by each other volume.
Now we have a number that means something to us. We can take that number and multiply it by the charge strength at the particle boundary and get the charge strength at that volume. We will actually use the 1/r^3 equation to find the value for any radius. I did think about using a lookup table like this but the equation isn't that expensive so it isn't worth it. Precision is more important.
So my initial guess of the maximum charge emission range being 10 radii is pretty good. At that distance, the charge strength should be 1000 times less than at the particle boundary. Is it a coincidence that the Earth's chargepause, which sets the magnetopause and others, is also at 11 to 12 radii? I think not. In fact, I know not because it is the same reasons for both. If I had realised that when I created the constant in the code I would have used 11 or 12 instead.
So now we have an equation to calculate the charge at a given distance: C(d) = C(1)/d^3
Please note that I am using an equation within an equation. C is an equation to calculate the charge density or strength given a radius. So that equation explicitly states: The charge density at d is equal to the charge density at 1 divided by d^3. The charge density equation could be as simple as mass times volume, maybe mass times surface area or it could be more convoluted.
This is a quantum equation. It does not apply to macro-scale numbers. We are ignoring time because the particles are close enough to do so, stars, planets, and moons are not, so it becomes a d^4 equation instead.
I have been playing with numbers again, and I think I see a way to calculate the charge strengths we want. Instead of a 1/r^4 calculation, it will be a 1/r^3 based equation. That is because it only uses the reduction in volume associated with that distance and not the time, which would bring in the distance again and give us r^4.
Analysis of Volume
Volume of a Sphere: (4*Pi*r^3)/3
Radius | Volume |
1 | 4.1887902048 |
2 | 33.5103216383 |
3 | 113.0973355292 |
4 | 268.0825731063 |
5 | 523.5987755983 |
6 | 904.7786842339 |
7 | 1436.7550402417 |
8 | 2144.6605848506 |
9 | 3053.6280592893 |
10 | 4188.7902047864 |
A radius of 1 represents the boundary of the emitter. That is the highest charge strength or charge density, which ever way you want to look at it.
A radius of 10 represents the furthest point away from the emitter in this dataset. Note that the radius is from the center of the emitter, not the boundary.
What we are looking at here is how and why the charge diminishes with distance. The particle only emits a certain amount of charge, at any given time or over some set time period, and that is constant (for our purposes here, it can change if the ambient field density changes but would still be a constant for us as we are working relative to the ambient field). So as that charge moves away from the particle, it inhabits a larger volume. The same amount of charge in a greater volume means less density.
So we want to look at how that volume changes as we use a greater radius. You can perform a little bit of calculus on it and find that the 3rd derivative, which is the constant derivative, has a value of 25.1327412287. That doesn't really tell us anything though. So let's take some ratios and see what it tells us.
Firstly, we will divide each volume by the first volume to see how many of the initial volume can fit into the larger volumes. In essence, this is making all volumes relative to the first volume.
Radius | Volume | V(n) / V(1) |
1 | 4.1887902048 | 1 |
2 | 33.5103216383 | 8 |
3 | 113.0973355292 | 27 |
4 | 268.0825731063 | 64 |
5 | 523.5987755983 | 125 |
6 | 904.7786842339 | 216 |
7 | 1436.7550402417 | 343 |
8 | 2144.6605848506 | 512 |
9 | 3053.6280592893 | 729 |
10 | 4188.7902047864 | 1000 |
A few of those numbers look familiar, and a quick analysis shows that each value is r^3. That wasn't too surprising, since we are using an r^3 equation, but I thought it worth showing as a stepping stone to the next part of this analysis.
That number tells us how much charge we could fit into each volume if we wanted to keep the charge density constant. But we don't want to keep the density constant, we want to keep the amount of charge constant and allow the density to change. So we are looking for the inverse of that value. We want to know how a given amount of charge will fit into a larger volume so we want to divide the first volume by each other volume.
Radius | Volume | V(n) / V(1) | V(1) / V(n) |
1 | 4.1887902048 | 1 | 1 |
2 | 33.5103216383 | 8 | 0.125 |
3 | 113.0973355292 | 27 | 0.037037037 |
4 | 268.0825731063 | 64 | 0.015625 |
5 | 523.5987755983 | 125 | 0.008 |
6 | 904.7786842339 | 216 | 0.0046296296 |
7 | 1436.7550402417 | 343 | 0.0029154519 |
8 | 2144.6605848506 | 512 | 0.001953125 |
9 | 3053.6280592893 | 729 | 0.0013717421 |
10 | 4188.7902047864 | 1000 | 0.001 |
Now we have a number that means something to us. We can take that number and multiply it by the charge strength at the particle boundary and get the charge strength at that volume. We will actually use the 1/r^3 equation to find the value for any radius. I did think about using a lookup table like this but the equation isn't that expensive so it isn't worth it. Precision is more important.
So my initial guess of the maximum charge emission range being 10 radii is pretty good. At that distance, the charge strength should be 1000 times less than at the particle boundary. Is it a coincidence that the Earth's chargepause, which sets the magnetopause and others, is also at 11 to 12 radii? I think not. In fact, I know not because it is the same reasons for both. If I had realised that when I created the constant in the code I would have used 11 or 12 instead.
So now we have an equation to calculate the charge at a given distance: C(d) = C(1)/d^3
Please note that I am using an equation within an equation. C is an equation to calculate the charge density or strength given a radius. So that equation explicitly states: The charge density at d is equal to the charge density at 1 divided by d^3. The charge density equation could be as simple as mass times volume, maybe mass times surface area or it could be more convoluted.
This is a quantum equation. It does not apply to macro-scale numbers. We are ignoring time because the particles are close enough to do so, stars, planets, and moons are not, so it becomes a d^4 equation instead.
Re: Possible Charged Particle Field
I haven't thought this too far through yet, and I might be shooting my mouth off before I see it clearly enough, but I am hesitantly going to say that I don't think the macro equation should be an r^4 equation either. I think that I have the correct equation above.
It needs to be changed for use in the macro setting, though. We need to include the change in size of the emitter. Since the receiver is so far away, the charge that it receives was emitted at a time in the past. Using expansion theory, this means that the radius of the emitter was smaller when it emitted that charge. Well, my equation has a radius for the emitter right there. So let's use it for this purpose and see what happens.
C(d) = C(1)/d^3
That tells us the reduction in charge density based on volume only. The radius of the emitter is used in 2 places, although only one of them actually represents the emitter radius.
The d variable is used to represent the distance of the receiver from the emitter. It should be the distance of the point of measurement from the center of the emitter. This is likely to be the boundary of the receiver from the center of the emitter.
The other place it is used is as the number 1. In this case, it represents the radius of the emitter. Before, we only needed the number 1 as everything was relative and based on 1. Now, we want to change that value so we make it a variable. Let's call it r, for radius of the emitter.
C(d) = C(r)/d^3
Now we just need to know what r was when the charge was emitted. To find that, we need the distance it has traveled, which is d, since we know that it had a velocity of c, then we can determine how long it took that photon to travel to this point.
v = d/t -> vt = d -> t = d/v
v = c = 300, 000, 000m/s
t = d/c
Now we need to know how much a particle expands, per unit time, or unit time squared if it is an acceleration. Unfortunately, I don't know what that is. I don't even have a good guess. Maybe we can tease it out but I'll use a placeholder here instead. I'll create another equation for it and we can fill it in later.
Let R(n) be a function that calculates the radius of a sphere, given a value, n, that represents the time difference. When n=0, the current radius is returned. When n>0, a future radius is returned with n being the number of seconds into the future. When n<0, a past radius is returned with (the absolute value of) n being the number of seconds into the past.
Therefore, putting it back into our equation, we get:
C(d) = C( R(-d/c) )/d^3
That is a general equation that can be used at the micro or macro scale, but more useful at the macro. Maybe you could call it a relativistic equation, since it takes Relativity into account. It does contain 2 other equations, or functions, that need to be provided in order to find the actual equation.
Not a bad days work, eh?
It needs to be changed for use in the macro setting, though. We need to include the change in size of the emitter. Since the receiver is so far away, the charge that it receives was emitted at a time in the past. Using expansion theory, this means that the radius of the emitter was smaller when it emitted that charge. Well, my equation has a radius for the emitter right there. So let's use it for this purpose and see what happens.
C(d) = C(1)/d^3
That tells us the reduction in charge density based on volume only. The radius of the emitter is used in 2 places, although only one of them actually represents the emitter radius.
The d variable is used to represent the distance of the receiver from the emitter. It should be the distance of the point of measurement from the center of the emitter. This is likely to be the boundary of the receiver from the center of the emitter.
The other place it is used is as the number 1. In this case, it represents the radius of the emitter. Before, we only needed the number 1 as everything was relative and based on 1. Now, we want to change that value so we make it a variable. Let's call it r, for radius of the emitter.
C(d) = C(r)/d^3
Now we just need to know what r was when the charge was emitted. To find that, we need the distance it has traveled, which is d, since we know that it had a velocity of c, then we can determine how long it took that photon to travel to this point.
v = d/t -> vt = d -> t = d/v
v = c = 300, 000, 000m/s
t = d/c
Now we need to know how much a particle expands, per unit time, or unit time squared if it is an acceleration. Unfortunately, I don't know what that is. I don't even have a good guess. Maybe we can tease it out but I'll use a placeholder here instead. I'll create another equation for it and we can fill it in later.
Let R(n) be a function that calculates the radius of a sphere, given a value, n, that represents the time difference. When n=0, the current radius is returned. When n>0, a future radius is returned with n being the number of seconds into the future. When n<0, a past radius is returned with (the absolute value of) n being the number of seconds into the past.
Therefore, putting it back into our equation, we get:
C(d) = C( R(-d/c) )/d^3
That is a general equation that can be used at the micro or macro scale, but more useful at the macro. Maybe you could call it a relativistic equation, since it takes Relativity into account. It does contain 2 other equations, or functions, that need to be provided in order to find the actual equation.
Not a bad days work, eh?
Re: Possible Charged Particle Field
I think that equation is a bit misleading. It seemed a good way to represent it as I wrote it, but now I see that the C(d) part is a bit confusing because the same function is used on the other side of that equation but it turns out that they are not really the same function.
What I should say is that the left hand side, C(d), is not actually a function call, but on the right hand side, C(1) or C( R(-d/c) ), it is a function call. That is because the right hand side is the implementation of the left hand side. Which means that they are not really the same function.
So let's clear that up.
Let:
R(t) be a function that must return the radius of a particle given a time differential, t, where t is in unit-time, for example, the number of seconds. When t=0, the current radius is returned. When t>0, a future radius is returned. When t<0, a past radius is returned.
C(r) be a function that returns the charge density at the boundary of a particle with radius r.
D(d) be a function that returns the charge density of a particle, measured at a distance, d, from its center.
c be the speed of light.
Therefore, the equation becomes:
D(d) = C( R( -d/c ) ) / d^3
I have also had some thoughts on the function C. If my memory serves me, then Miles has stated that the charge density of a body (he was talking about stars, planets and moons) is its mass times by its density. Quite literally a charge density or mass density. I am not so sure that I want the same equation because that assumes you want the total charge of the body. I don't really want that, although I could work with it.
You see, we are not only trying to find the charge density at a distance, we are also trying to find the charge density over a certain area which is defined by the receiver. So far, we have not taken the receiver into account at all but it is part of this interaction and so it must be represented.
To combat that, I thought the charge density function, C, could return the mass, of the emitter, divided by its surface area. This gives us a charge density per unit area. Now, this unit area is small on the emitter, but it could be extremely large at the receiver. How do we deal with that?
Well, we could not do what I have just mentioned (divide by the emitters surface area) and instead, we calculate the total charge output of the emitter, for a complete sphere, reduce it based on the distance to the receiver, and then divide it by the surface area of the sphere that is centered on the emitter, but with a radius equal to the distance of the measurement point (receiver). If the receiver is a volume, rather than a point, then we might multiply that result by the surface area of the receiver (or maybe half of it) to find the total charge density across the receiver.
I think this is very important stuff. I have not only found an equation that sits along side Einsteins' equations, which he had no way of knowing was needed, but I have also found a way to use Expansion Theory without actually expanding the radii of particles. In fact, I am really using it to contract the radii, but only when calculating a charge density, not during the normal run of the model.
If I can find a way to do the same thing with gravity, I will have the basis of an Expansion Model.
What I should say is that the left hand side, C(d), is not actually a function call, but on the right hand side, C(1) or C( R(-d/c) ), it is a function call. That is because the right hand side is the implementation of the left hand side. Which means that they are not really the same function.
So let's clear that up.
Let:
R(t) be a function that must return the radius of a particle given a time differential, t, where t is in unit-time, for example, the number of seconds. When t=0, the current radius is returned. When t>0, a future radius is returned. When t<0, a past radius is returned.
C(r) be a function that returns the charge density at the boundary of a particle with radius r.
D(d) be a function that returns the charge density of a particle, measured at a distance, d, from its center.
c be the speed of light.
Therefore, the equation becomes:
D(d) = C( R( -d/c ) ) / d^3
I have also had some thoughts on the function C. If my memory serves me, then Miles has stated that the charge density of a body (he was talking about stars, planets and moons) is its mass times by its density. Quite literally a charge density or mass density. I am not so sure that I want the same equation because that assumes you want the total charge of the body. I don't really want that, although I could work with it.
You see, we are not only trying to find the charge density at a distance, we are also trying to find the charge density over a certain area which is defined by the receiver. So far, we have not taken the receiver into account at all but it is part of this interaction and so it must be represented.
To combat that, I thought the charge density function, C, could return the mass, of the emitter, divided by its surface area. This gives us a charge density per unit area. Now, this unit area is small on the emitter, but it could be extremely large at the receiver. How do we deal with that?
Well, we could not do what I have just mentioned (divide by the emitters surface area) and instead, we calculate the total charge output of the emitter, for a complete sphere, reduce it based on the distance to the receiver, and then divide it by the surface area of the sphere that is centered on the emitter, but with a radius equal to the distance of the measurement point (receiver). If the receiver is a volume, rather than a point, then we might multiply that result by the surface area of the receiver (or maybe half of it) to find the total charge density across the receiver.
I think this is very important stuff. I have not only found an equation that sits along side Einsteins' equations, which he had no way of knowing was needed, but I have also found a way to use Expansion Theory without actually expanding the radii of particles. In fact, I am really using it to contract the radii, but only when calculating a charge density, not during the normal run of the model.
If I can find a way to do the same thing with gravity, I will have the basis of an Expansion Model.
Re: Possible Charged Particle Field
I know I am getting pretty far off track, but this is so important that I just need to get it out. I need to run with it while it is fresh in my mind.
Re: Possible Charged Particle Field
Note the differences between the quantum equation and the relativistic one.
1) D(d) = C(1)/d^3
2) D(d) = C( R( -d/c ) )/d^3
Equation 1 only has the value 1 inside of the C function call. This represents the current radius of the emitter.
Equation 2 has R( -d/c ) inside of the C call, which represents the radius of the emitter at some time in the past.
If we look at the definition of the R function, we find that when its argument is equal to 0, it will return the current radius.
When d is extremely small, the ratio of d to c approaches 0. It will never actually equal 0, but it will get so close as to be irrelevant.
Therefore, the relativistic equation reduces to the quantum equation at small distances.
1) D(d) = C(1)/d^3
2) D(d) = C( R( -d/c ) )/d^3
Equation 1 only has the value 1 inside of the C function call. This represents the current radius of the emitter.
Equation 2 has R( -d/c ) inside of the C call, which represents the radius of the emitter at some time in the past.
If we look at the definition of the R function, we find that when its argument is equal to 0, it will return the current radius.
When d is extremely small, the ratio of d to c approaches 0. It will never actually equal 0, but it will get so close as to be irrelevant.
Therefore, the relativistic equation reduces to the quantum equation at small distances.
Re: Possible Charged Particle Field
.
You've definitely hit something, you've got to run with it. Including gravity would win you a seat beside Miles. The only thing I see missing is spin, then you can combine it with your angular motion and spin level velocity formulas.
Being critical, I might point out that emission fields aren’t spherical. The charged particle’s emission field is planar, like a disc, not a sphere. Actually, I would insist that a particle’s emission field may be better described as dual discs - due to the separate +/-30deg emissions. Or a neutron’s polar charge emissions have the least amount of energy since it receives no angular emission from the spinning particle, what’s the charge density range for polar emissions? My point is the particle’s emission field is more complicated than a spherical volume. I’ve insisted charge emission is a function of latitude. When we add the components necessary in order to arrive at a complete description of the particle’s emission field we may end up with a charge lookup table.
Good stuff Nevyn.
.
Ok, I'll try to read them. The charge density felt at distance d, depends on the emitter’s radius and the change in the emitter’s size in the time it took for light to reach the distance being measured, relativistic charge emission. -d/c approaches 0 for the quantum scale. Together, both equations combine the micro or macro scales based on an analysis of spherical volume. It reminds me of the fact that spherical surface area is used to describe the inverse relationship of gravity, how after doubling a radius, what was a unit area becomes an area four time as large. Not a bad days work at all. More like an epiphany, the particle scaling relationship you’ve been looking for.Quantum. D(d) = C(1)/d^3
Relativistic. D(d) = C( R( -d/c ) )/d^3
You've definitely hit something, you've got to run with it. Including gravity would win you a seat beside Miles. The only thing I see missing is spin, then you can combine it with your angular motion and spin level velocity formulas.
Being critical, I might point out that emission fields aren’t spherical. The charged particle’s emission field is planar, like a disc, not a sphere. Actually, I would insist that a particle’s emission field may be better described as dual discs - due to the separate +/-30deg emissions. Or a neutron’s polar charge emissions have the least amount of energy since it receives no angular emission from the spinning particle, what’s the charge density range for polar emissions? My point is the particle’s emission field is more complicated than a spherical volume. I’ve insisted charge emission is a function of latitude. When we add the components necessary in order to arrive at a complete description of the particle’s emission field we may end up with a charge lookup table.
Good stuff Nevyn.
.
LongtimeAirman- Admin
- Posts : 2034
Join date : 2014-08-10
Re: Possible Charged Particle Field
LongtimeAirman wrote:
You've definitely hit something, you've got to run with it. Including gravity would win you a seat beside Miles.
I think including gravity will earn me a seat next to Einstein. Maybe even above Einstein! I'm not trying to make myself sound great or anything, there's still so much I don't know, but if I do that, then I have actually done what Einstein said that he did, but didn't quite get it correct, or at least complete.
But I'm still doubting myself at this point. I keep re-reading it to make sure I haven't missed anything or buggered something up. I don't care about being compared to Einstein, just that we figure out Physics before it's too late. I am standing on the shoulders of both Miles and Einstein here, and give that credit where it is due. There is absolutely no way that I would have reached this position without Miles work behind me. None at all.
LongtimeAirman wrote:
The only thing I see missing is spin, then you can combine it with your angular motion and spin level velocity formulas.
Ah, but as we have already implemented in this app, spin comes from measuring the charge interactions from multiple places on the receiver and applying them in the correct fashion. We've already completed that step!
LongtimeAirman wrote:
Being critical, I might point out that emission fields aren’t spherical. The charged particle’s emission field is planar, like a disc, not a sphere. Actually, I would insist that a particle’s emission field may be better described as dual discs - due to the separate +/-30deg emissions. Or a neutron’s polar charge emissions have the least amount of energy since it receives no angular emission from the spinning particle, what’s the charge density range for polar emissions? My point is the particle’s emission field is more complicated than a spherical volume. I’ve insisted charge emission is a function of latitude. When we add the components necessary in order to arrive at a complete description of the particle’s emission field we may end up with a charge lookup table.
Totally true and well done for seeing it so quickly. However, we have already fixed that problem in this app too! My charge density algorithm, already in use, takes care of the latitude differences. That algorithm would be inside of the C function used in those equations. It does need more work, though, especially around the polar regions. It would also be nice if I could reduce it to an equation rather than an algorithm. I'm not sure that is possible.
My main concern at this point is the R function. I'm just not sure how to go about implementing that. While I have some idea of what the C function looks like, figuring out the radius changes is a bit more difficult. I am hoping that I find that gravity is not really a 1/r^2 relationship. I am hoping that I find the same thing I did here, that one of those distances is actually a time separation and will not be required in the math, in that way. Miles has already said as much, but I don't think he has taken that concept down to the equations like I am trying to here.
To bring this back down to Earth, and demonstrate that I don't have an expanding ego, all I am doing here is analyzing geometry. I decided to go back to basics to determine how charge diminishes so that I could find the spot that I needed to change it, and have found a few things in the process. I am not doing anything that would be considered great, or of high intelligence. How many times has Miles said that science has gotten so far ahead of itself and just needs to get back to basics? And again we find it here. Physics doesn't need intelligence, it only needs mechanics. When you have the mechanics, the math just falls out of it. The mechanics controls the math. It provides the boundaries that the math must follow. It gives you the concepts that the math must implement.
So the next time you hear a Physicist say that the 'math is everything', you know they are lying. If the mechanics control the math, then the mechanics is more important than the math. That is because the mechanics represents reality and if your math is not tied to reality, then it is not Physics.
Re: Possible Charged Particle Field
Something else I would like to point out is that it is Expansion Theory that has allowed me to find this stuff. By reducing gravity to a changing radius, a real motion of real entities, Miles has provided the basis for the mechanics that I needed. All I did was notice that I needed a smaller radius to represent the past (again provided by Miles), and that I had a radius in such a position. So I ran with it and am pretty happy with where I have ended up.
There is still a lot of work required, but what I have found, in only a few hours, is a great start. I not only have the general structure of the equation, I also have the placeholders for future work. And they are not just undefined placeholders either, they are clearly defined. While I don't know their exact implementations right now, I do know what they need to do.
When was the last time you saw that in mainstream Physics?
Aaaaaand, my ego is expanding again. Does anyone have a pin?
There is still a lot of work required, but what I have found, in only a few hours, is a great start. I not only have the general structure of the equation, I also have the placeholders for future work. And they are not just undefined placeholders either, they are clearly defined. While I don't know their exact implementations right now, I do know what they need to do.
When was the last time you saw that in mainstream Physics?
Aaaaaand, my ego is expanding again. Does anyone have a pin?

Re: Possible Charged Particle Field
Jesus, Nevyn.
I'll be that pin, but only because you've been my pin in the past. Consider it returning the favor!
But this is some good shit right here. I'm not at all familiar with the ins and outs of the app or the script you're using, but ALL of the expansion equations here and your explanation makes sense to me. You're attacking it in a way that would have never occurred to me. It's definitely what you think, an extension of Miles and Einstein and all the other works but in a revolutionary way here. I think you need to keep going, and only wish I had the capacity to help aside from cheerleading. But it's something I might be able to plop into Maya as well, since it's so straightforward! Perhaps all of this scripting can be fairly easily converted to Python or Pymel, and save me all the headaches I've had with the Ndynamics "Nucleus" solver (ironic since it can't handle an actual nucleus!).
Allow yourself some patience though too! But as is your wont, run with it as far and fast as you can. You can always refine it later.
I'll be that pin, but only because you've been my pin in the past. Consider it returning the favor!
But this is some good shit right here. I'm not at all familiar with the ins and outs of the app or the script you're using, but ALL of the expansion equations here and your explanation makes sense to me. You're attacking it in a way that would have never occurred to me. It's definitely what you think, an extension of Miles and Einstein and all the other works but in a revolutionary way here. I think you need to keep going, and only wish I had the capacity to help aside from cheerleading. But it's something I might be able to plop into Maya as well, since it's so straightforward! Perhaps all of this scripting can be fairly easily converted to Python or Pymel, and save me all the headaches I've had with the Ndynamics "Nucleus" solver (ironic since it can't handle an actual nucleus!).
Allow yourself some patience though too! But as is your wont, run with it as far and fast as you can. You can always refine it later.
Jared Magneson- Posts : 525
Join date : 2016-10-11
Page 3 of 15 • 1, 2, 3, 4 ... 9 ... 15

» Particle Drifts in Space
» Modeling a Charge Particle
» Spinning Particle Language
» The First Ever Photograph of Light as both a Particle and Wave
» Physicists Finally Narrowed Down the Mass of the Tiniest 'Ghost Particle' in the Universe
» Modeling a Charge Particle
» Spinning Particle Language
» The First Ever Photograph of Light as both a Particle and Wave
» Physicists Finally Narrowed Down the Mass of the Tiniest 'Ghost Particle' in the Universe
Page 3 of 15
Permissions in this forum:
You cannot reply to topics in this forum
|
|